
圖片來源: 網路
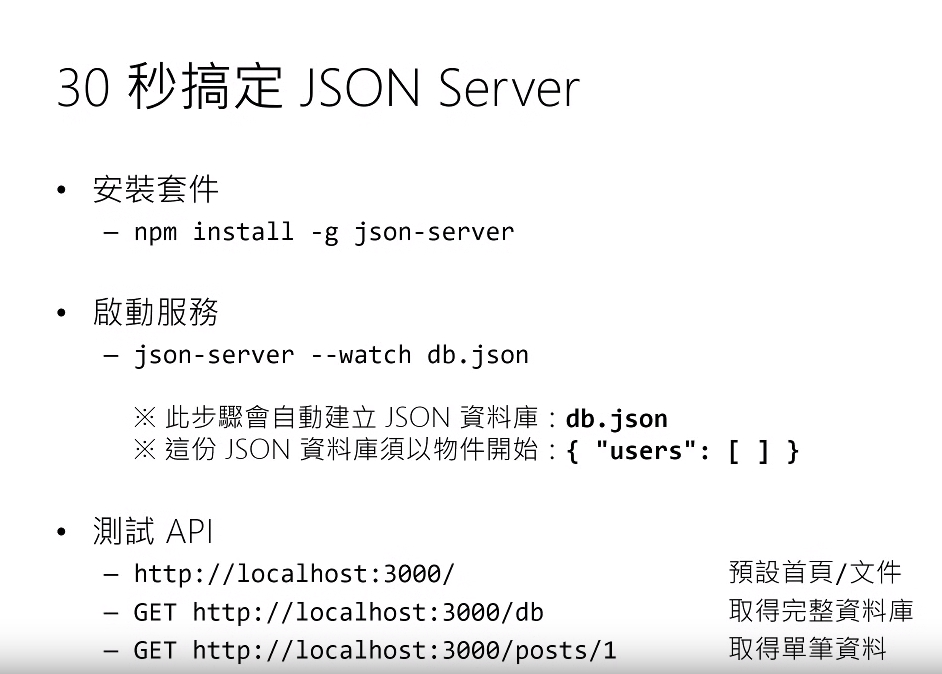
圖片來源: 2017-05-30 (直播錄影) 進擊的前端工程師:今天就自己打造 API 吧! (高清版)
# 教學影片資源
[ Alex 宅幹嘛 ] 👨💻 JSON 與 RESTFUL API 串接一次搞定 ( JSON Server + Postman Chrome 瀏覽器 API 測試外掛 + jQuery )
[ Alex 宅幹嘛 ] 👨💻Vue 全家桶與 RESTful API 串接入門介紹 (JSON Server + Vue + axios)
【2019/5/3】 基本 AJAX 請求,練習 CRUD
下載 JSON server
npm install -g json-server
新增 json 檔案
隨意新增一些資料,內容自訂,並存成 .json
檔案
用 json server 監控該檔案
json-server --watch db.json
HTTP 請求基本功能
index.html
內撰寫的 JavaScript 來對該資料庫進行CRUD
(新增刪除修改)
程式碼
- json
1 | { |
HTML
1 | <div id="create"> |
JavaScript
1 | $(function () { |